This section explains how to implement Toppers/ASP3 in the same environment as the CARTOS section, so please refer to it as well.
Read
Event flags
I will also briefly mention event flags.
In the case of event flags, we use T_CFLG
.
T_CFLG flg; flg.flgatr = TA_CLR; flg.iflgptn = 0;
Generating an Event Flag
fid = acre_flg(&flg);
Set the event flag
set_flg(fid, FLG_PTN);
※FLG_PTN
is any 32-bit length
To use an event flag in a task, wait for the event flag to be set in wai_flg
.
Example of Event Flags Usage
void adc_task(intptr_t exif) { FLGPTN flg_ptn; /* ToDo */ for(;;) { wai_flg(fid, FLG_PTN, TWF_ANDW, &flg_ptn); clr_flg(fid, 0); } }
When waiting for an event flag, you must define FLGPTN flg_ptn
beforehand to be able to receive the flag pattern. Then, wai_flg(...)
must be able to receive the flag pattern (FLG_PTN)
from the target event flag ID (fid)
with the specified pattern (FLG_PTN)
and the received flag pattern (flg_ptn You can only execute the next line if (TWF_ANDW)
is an exact match (TWF_ANDW)
. Otherwise, the process is moved on to another task. (This is usually referred to as being “dispatched”.)
Also, if the pattern is matched by wai_flg()
, we need to clear the pattern in the received flag. clr_flg(fid, 0);
to clear the event flags and, in the case of this example task, to wait for the event flags again.
Figure 1 shows the RTOS viewer when the program is stopped when the event flag is set. You can see that the flag pattern has been set to 0x5555AAA
.
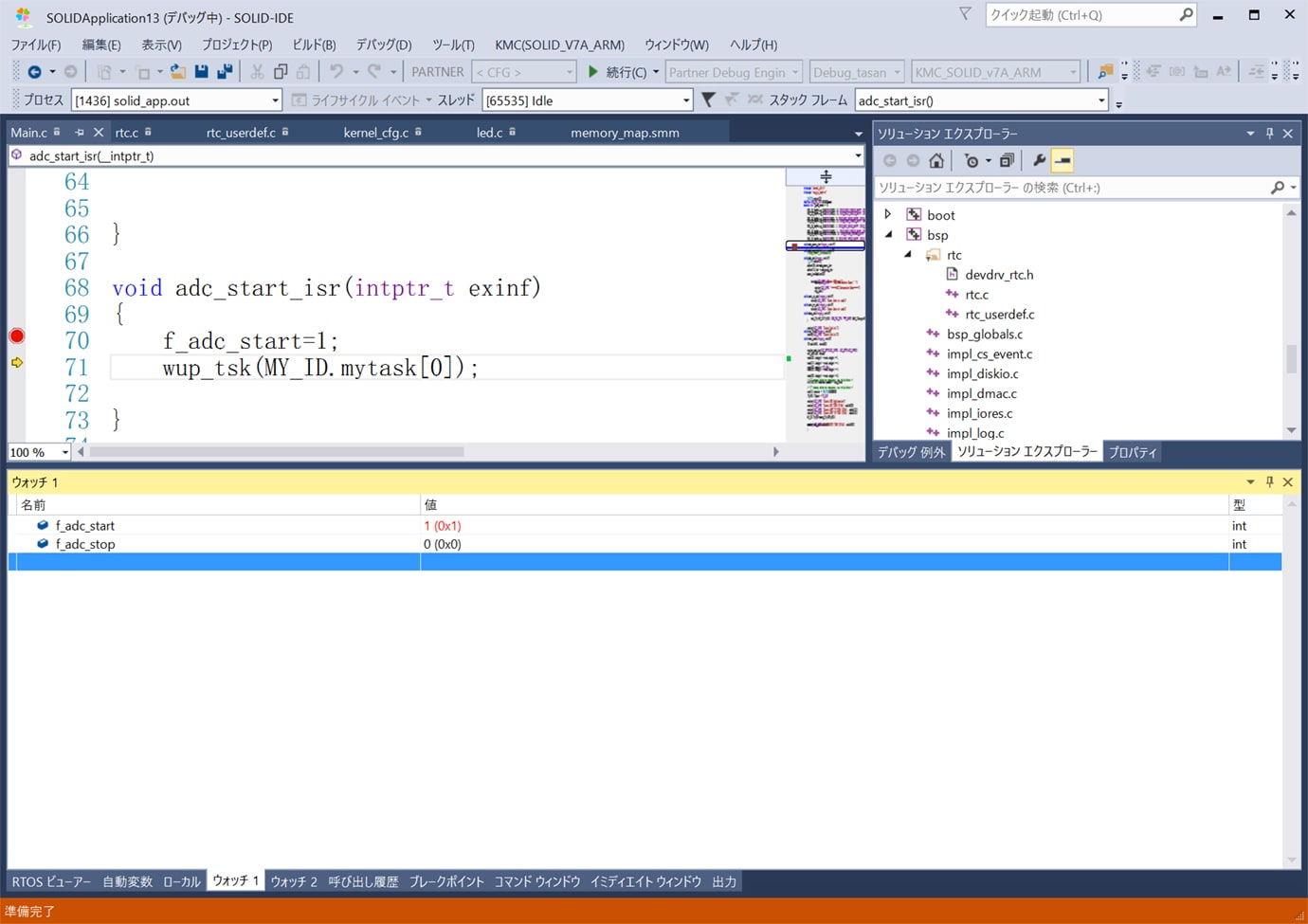
Figure 1: Display the status of the event flags.
Setting the Periodic Handler
The following is a brief description of the cyclic handlers.
Registration of Periodic Handler
For cyclic handlers, we use T_CCYC
and T_NFYINFO
. This example invokes a cyclic handler called cyc_func
every 10 seconds.
T_CCYC cyc; T_NFYINFO nfy; nfy.nfymode = 0; nfy.nfy.handler.tmehdr = cyc_func; cyc.cycatr = TA_NULL; cyc.nfyinfo = nfy; cyc.cyctim = (RELTIM)10000000; cyc.cycphs = (RELTIM)10000000; /** The body of the periodic handler is written like a function in the same way as a task. */ void cyc_func(intptr_t exif) { /* ToDo */ }
Generation of periodic handlers
The periodic handler is created by acre_cyc
.
cycid = acre_cyc(&cyc);
Invoke periodic handler
The periodic handler is invoked by sta_cyc
.
ercd = sta_cyc(cycid);
Figure 2 shows the state of the cyclic handler in the RTOS viewer.
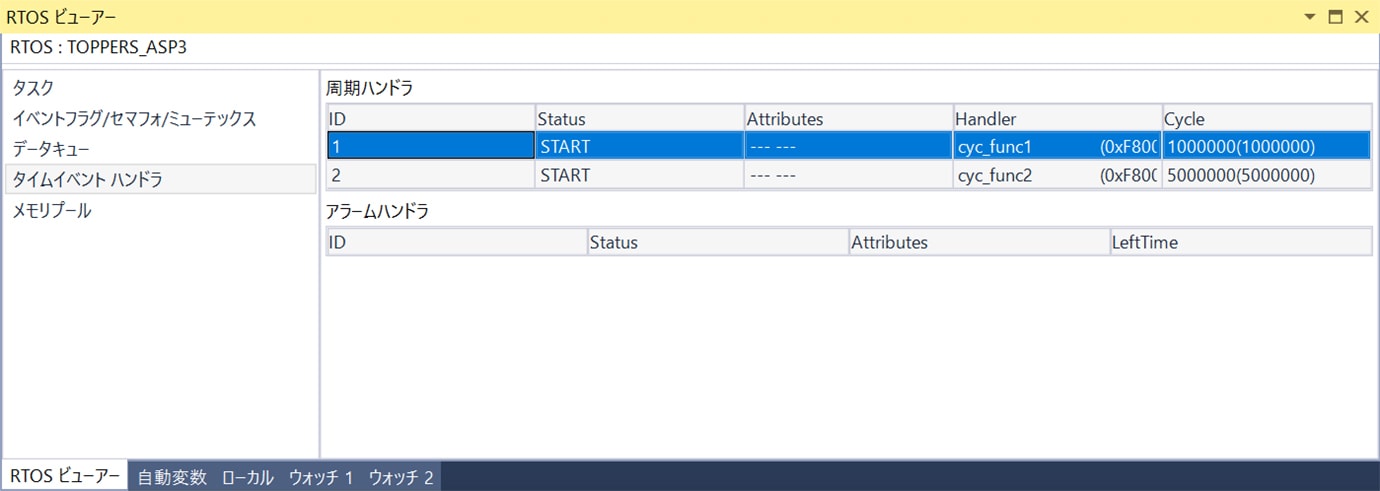
Figure 2: State of the periodic handler
setting interrupts
It is not an exaggeration to say that interrupts are used in almost all systems, no matter how fast the CPU is. They are used in many embedded systems.
SOLID-OS interrupts are based on Toppers/ASP3, which handles interrupts under the control of the kernel, and are sometimes called “interrupt service routines (ISRs)” or “interrupts in management”. The others are called “interrupt handler” or “kernel unmanaged interrupts”.
Interrupt Vector Table
When you use interrupts in an embedded system, you’ll have to fiddle with a few files. One of them is called an interrupt vector table.
In SOLID-OS, it’s basically the same idea, but you can register it in kernel_cfg.c
. Let’s take a look at this in practice.
Register the number of interrupt resources to be used, defined in kernel_cfg.c as follows.
#define TNUM_AID_ISR 0 //ISR(interrupt service routine
When two ISRs are used, they are defined as follows
#define TNUM_AID_ISR 2 //ISR(interrupt service routine
Interrupt initialization configuration block
Next, we will set the “Interrupt Initialization Configuration Block”. In the case of RZ/A, the interrupt IDs correspond to the interrupt IDs: GPI_2
becomes 34
, GPI_3
becomes 35
, and so on. It is.
const INTINIB _kernel_intinib_table[] = { {34, TA_NULL, INTINIB_USE_ISR, TA_NULL, -16}, {35, TA_NULL, INTINIB_USE_ISR, TA_NULL, -16}, }
Register with this content. If you want to use other interrupt sources, change the interrupt ID. SOLID-OS is very convenient because you can write it in kernel_cfg.c
.
Register an interrupt service routine
The next step is to register the interrupt service routine. Use T_CISR
.
T_CISR adisr[2]; adisr[0].isr = adc_start_isr; adisr[0].intno = 34; adisr[0].isratr = TA_NULL; adisr[0].isrpri = 2; adisr[1].isr = adc_stop_isr; adisr[1].intno = 35; adisr[1].isratr = TA_NULL; adisr[1].isrpri = 2;
What is registered in adisr[0]
is the setting for when the “start button” is used as an interrupt, which is assigned to GPI_2
. And since the priority of the interrupt is relatively high, we have set it to 2
. The function corresponding to the interrupt service routine is set to “adc_start_isr
“.
adc_start_isr
is as follows.
void adc_start_isr(intptr_t exif) { /* Flag setting */ /* Launching a Waiting Task*/ wup_tsk(…); }
Similarly, adisr[1]
is registered as a “stop button” and GPI_3
is assigned to it. The function of the interrupt service routine is “adc_stop_isr
“.
adc_stop_isr
is as follows.
void adc_start_isr(intptr_t exif) { /* Flag setting */ }
The flag setting here is not an event flag, but just a flag on a global variable (Figure 3). Of course, you can also specify an event flag.
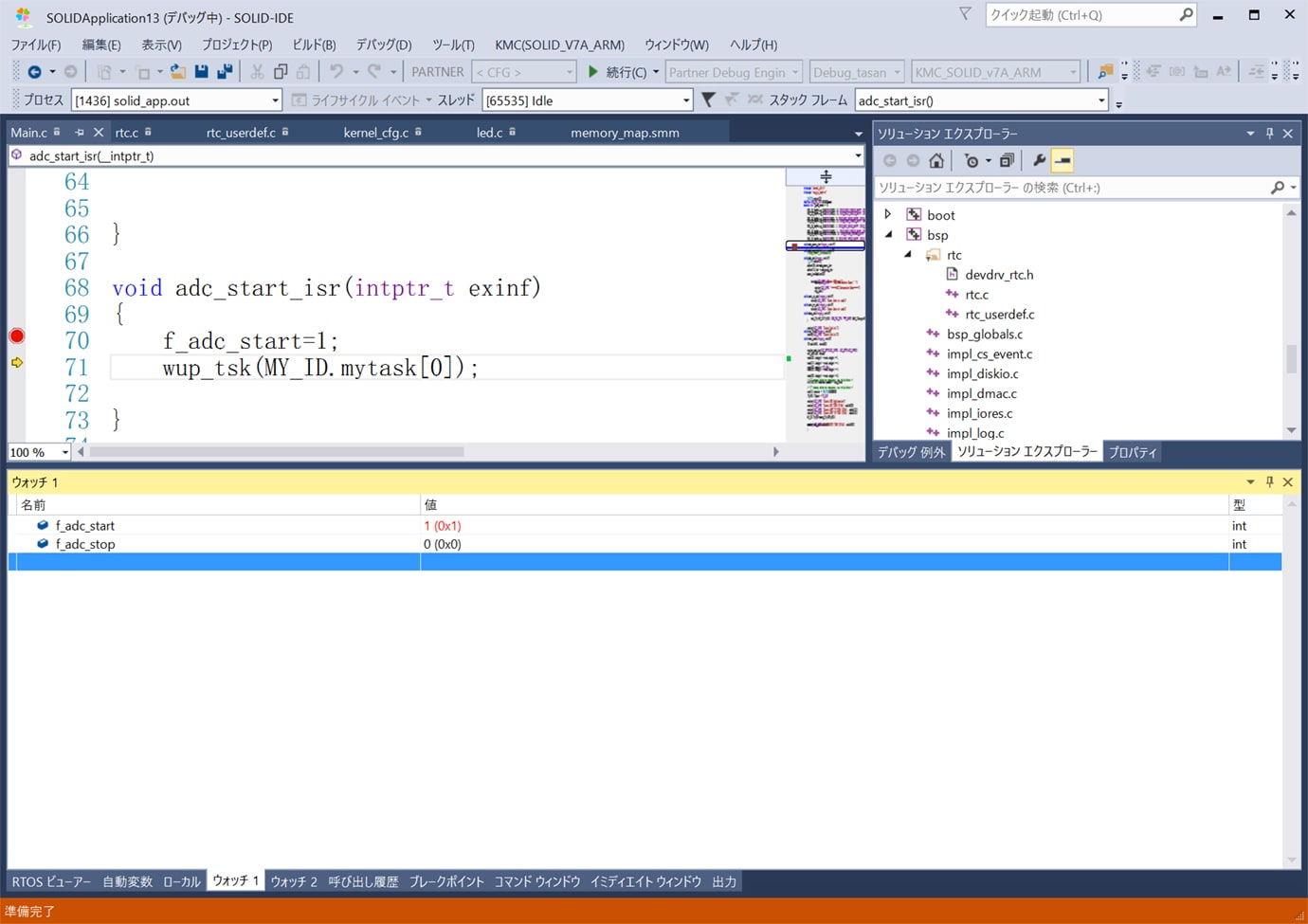
Figure 3: Flag operation by the interrupt interruption service routine
Even in the same µITRON system, when a task is controlled by an interrupt service routine, some of them should be iwup_tsk
, but in the case of Toppers/ASP3, they are treated as the same.
Generate an interrupt service routine
Once the interrupt has been registered, an interrupt service routine must be generated.
acre_isr(&adisr[0]); acre_isr(&adisr[1]);
The interrupt service routine is now registered under kernel management.
Allow and disallow interrupts
In order for an interrupt service routine to work, interrupts must be allowed.
Allow and Disallow Interrupts
- allowing for interruptions
ena_int(…)
- no intervention
dis_int(…)
The argument must include the appropriate interrupt number (or interrupt ID in RZ/A). This will allow the button interrupt to work at any time. In this article, we have actually used SOLID-OS resources to touch on the use of tasks, event flags, periodic handlers, and even interrupt service routines.
“もっと見る” カテゴリーなし
Mbed TLS overview and features
In this article, I'd like to discuss Mbed TLS, which I've touched on a few times in the past, Transport …
What is an “IoT device development platform”?
I started using Mbed because I wanted a microcontroller board that could connect natively to the Internet. At that time, …
Mbed OS overview and features
In this article, I would like to write about one of the components of Arm Mbed, and probably the most …